Definition
Define a family of algorithms, encapsulate each one, and make them interchangeable. Strategy lets the algorithm vary independently from clients that use it.
UML class diagram
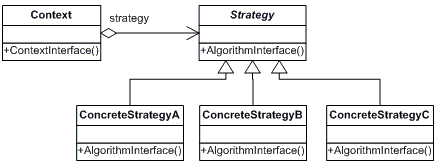
Participants
The classes and/or objects participating in this pattern are:
- Strategy - declares an interface common to all supported algorithms. Context uses this interface to call the algorithm defined by a ConcreteStrategy.
- ConcreteStrategy - implements the algorithm using the Strategy interface
is configured with a ConcreteStrategy object
maintains a reference to a Strategy object
may define an interface that lets Strategy access its data.
Sample code in C#
This allows clients to dynamically change sorting strategies including Quicksort, Shellsort, and Mergesort.
///
/// MainApp startup class for Real-World
/// Strategy Design Pattern.
///
class MainApp
{
///
/// Entry point into console application.
///
static void Main()
{
// Two contexts following different strategies
SortedList studentRecords = new SortedList();
studentRecords.Add("Samual");
studentRecords.Add("Jimmy");
studentRecords.Add("Sandra");
studentRecords.Add("Vivek");
studentRecords.Add("Anna");
studentRecords.SetSortStrategy(new QuickSort());
studentRecords.Sort();
studentRecords.SetSortStrategy(new ShellSort());
studentRecords.Sort();
studentRecords.SetSortStrategy(new MergeSort());
studentRecords.Sort();
// Wait for user
Console.ReadKey();
}
}
///
/// The 'Strategy' abstract class
///
abstract class SortStrategy
{
public abstract void Sort(List<string> list);
}
///
/// A 'ConcreteStrategy' class
///
class QuickSort : SortStrategy
{
public override void Sort(List<string> list)
{
list.Sort(); // Default is Quicksort
Console.WriteLine("QuickSorted list ");
}
}
///
/// A 'ConcreteStrategy' class
///
class ShellSort : SortStrategy
{
public override void Sort(List<string> list)
{
//list.ShellSort(); not-implemented
Console.WriteLine("ShellSorted list ");
}
}
///
/// A 'ConcreteStrategy' class
///
class MergeSort : SortStrategy
{
public override void Sort(List<string> list)
{
//list.MergeSort(); not-implemented
Console.WriteLine("MergeSorted list ");
}
}
///
/// The 'Context' class
///
class SortedList
{
private List<string> _list = new List<string>();
private SortStrategy _sortstrategy;
public void SetSortStrategy(SortStrategy sortstrategy)
{
this._sortstrategy = sortstrategy;
}
public void Add(string name)
{
_list.Add(name);
}
public void Sort()
{
_sortstrategy.Sort(_list);
// Iterate over list and display results
foreach (string name in _list)
{
Console.WriteLine(" " + name);
}
Console.WriteLine();
}
}
-
-
Letture(1709)
-
Permalink