Definition
Define a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
UML class diagram
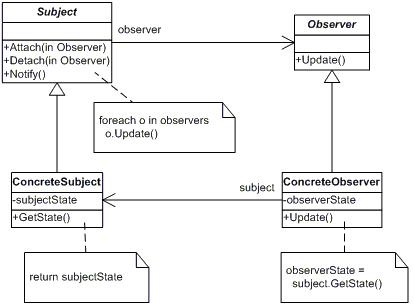
Participants
The classes and/or objects participating in this pattern are:
- Subject
provides an interface for attaching and detaching Observer objects.
- ConcreteSubject
sends a notification to its observers when its state changes
- Observer
*ConcreteObserver
maintains a reference to a ConcreteSubject object
stores state that should stay consistent with the subject's
implements the Observer updating interface to keep its state consistent with the subject's
Sample code in C#
#region Subject
public abstract class Subject
{
#region Members
private List_observers;
#endregion
#region Ctor
///
/// Construct a new subject object
///
public Subject()
{
_observers = new List();
}
#endregion
#region Methods
///
/// Attaches a new observer to the subject
///
/// The observer to attach
public void Attach(IObserver observer)
{
_observers.Add(observer);
}
///
/// Detaches an observer from the subject
///
/// The observer to detach
public void Detach(IObserver observer)
{
_observers.Remove(observer);
}
///
/// Notify all the observers about the change in the
/// subject's state
///
public void Notify()
{
foreach (IObserver observer in _observers)
{
observer.Update();
}
}
#endregion
}
#endregion
#region Concrete Subject
public class ConcreteSubject: Subject
{
#region Properties
///
/// The state of the subject
///
public T SubjectState { get; set; }
#endregion
}
#endregion
#region Observer
public interface IObserver
{
void Update();
}
#endregion
#region Concrete Observer
public class ConcreteObserver: IObserver
{
#region Properties
///
/// The subject the observer holds
///
public ConcreteSubjectSubject { get; set; }
private T _observerState;
#endregion
#region Ctor
///
/// Construct a new concrete observer with the given
/// subject
///
/// The given subject
public ConcreteObserver(ConcreteSubjectsubject)
{
Subject = subject;
}
#endregion
#region IObserver Members
///
/// Make an update to the observer state whenever the
/// method is callled
///
public void Update()
{
_observerState = Subject.SubjectState;
Console.WriteLine("The new state of the observer:{0}",
_observerState.ToString());
}
#endregion
}
#endregion
The example is simple to follow. We have an IObserver interface and a Subject abstract class. The observers are registered in the subject with the Attach method and also can be detached. The subject implement the Notify method that notifies every observer when the subject state was changed. When the state changes the observer make an update which is the main method of the IObserver interface.
The following code is an example scenario of how to run the pattern in console application:
ConcreteSubject<string> subject =new ConcreteSubject<string>();
subject.Attach(new ConcreteObserver<string>(subject));
subject.Attach(new ConcreteObserver<string>(subject));
subject.SubjectState = "Hello World";
subject.Notify();
Console.Read();
-
-
Leest(1827)
-
Permalink